Python OpenCV fullscreen webcam video : improve image quality
- Alibek Jakupov
- Nov 15, 2020
- 1 min read
Updated: Apr 18, 2024
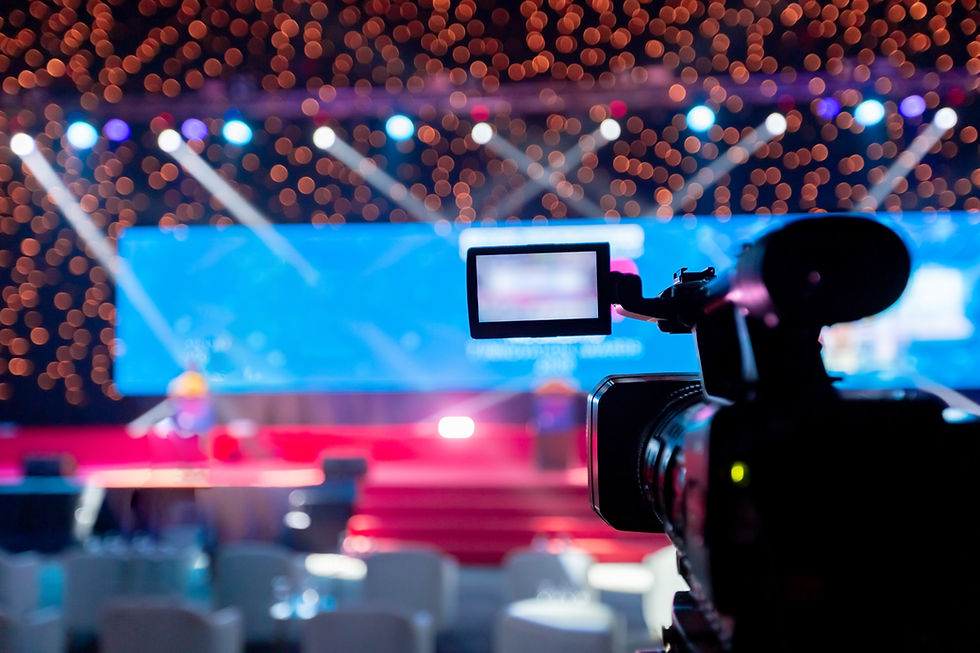
In one of the previous articles we've already discussed how to use exported Azure Custom Vision models with OpenCV in real time. However, you may have noticed that on Windows, passing to fullscreen mode results in a poor quality of graphical elements (even the text).
In this short article, you'll see the Rookie's approach to resolve the issue. Up we go!
Let's first of all have a look on the initial result.
If you remember to activate the full-screen mode you need to do the following:
# Full screen mode
cv2.namedWindow(WINDOW_NAME, cv2.WND_PROP_FULLSCREEN)
cv2.setWindowProperty(WINDOW_NAME, cv2.WND_PROP_FULLSCREEN, cv2.WINDOW_FULLSCREEN)
And, as the result, you see:
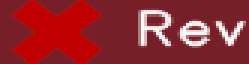
To compare, let's take a look at the output, in a non-fullscreen mode

You see that the curves are much less smooth, even the text is quite ugly.
So what I've tried to do, is to resize the image to fit the screen size and to activate full screen mode after.
Step 1: Get the screen dimensions
import ctypes
## get Screen Size
user32 = ctypes.windll.user32
screen_width, screen_height = user32.GetSystemMetrics(0), user32.GetSystemMetrics(1)
Step 2: Resize the frame
frame_height, frame_width, _ = frame.shape
scaleWidth = float(screen_width)/float(frame_width)
scaleHeight = float(screen_height)/float(frame_height)
if scaleHeight>scaleWidth:
imgScale = scaleWidth
else:
imgScale = scaleHeight
newX,newY = frame.shape[1]*imgScale, frame.shape[0]*imgScale
frame = cv2.resize(frame,(int(newX),int(newY)))
And after, activate the fullscreen mode. Here's the complete snippet
import cv2
import ctypes
WINDOW_NAME = 'Full Integration'
# initialize video capture object to read video from external webcam
video_capture = cv2.VideoCapture(1)
# if there is no external camera then take the built-in camera
if not video_capture.read()[0]:
video_capture = cv2.VideoCapture(0)
# Full screen mode
cv2.namedWindow(WINDOW_NAME, cv2.WND_PROP_FULLSCREEN)
cv2.setWindowProperty(WINDOW_NAME, cv2.WND_PROP_FULLSCREEN, cv2.WINDOW_FULLSCREEN)
while (video_capture.isOpened()):
# get Screen Size
user32 = ctypes.windll.user32
screen_width, screen_height = user32.GetSystemMetrics(0), user32.GetSystemMetrics(1)
# read video frame by frame
ret, frame = video_capture.read()
frame = cv2.flip(frame, 1)
frame_height, frame_width, _ = frame.shape
scaleWidth = float(screen_width)/float(frame_width)
scaleHeight = float(screen_height)/float(frame_height)
if scaleHeight>scaleWidth:
imgScale = scaleWidth
else:
imgScale = scaleHeight
newX,newY = frame.shape[1]*imgScale, frame.shape[0]*imgScale
frame = cv2.resize(frame,(int(newX),int(newY)))
cv2.imshow(WINDOW_NAME, frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# release video capture object
video_capture.release()
cv2.destroyAllWindows()
If we look at the output:
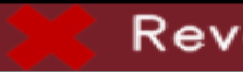
Slightly better, right?
I still continue my research to improve the image quality, but at Rookie developer blog, there's no such thing as a small improvement, each amelioration matters and worth sharing.
Hope someone will find it useful.
Comments